리액트에서 타입스크립트를 사용하여 props를 만들 때 타입스크립트 없이 만들 때와 가장 다른 점은 Child 컴포넌트 내부에서 어떤 props를 받을지 결정해야 한다는 것이다. 타입을 정하기 위해 컴포넌트(Child) 파일 내부에 인터페이스를 정의한다. 그 인터페이스는 Child 컴포넌트가 받아야 하는 props를 정의하는 것이다.
예를 들어 아래와 같은 두 개의 컴포넌트가 있고 Child 컴포넌트의 props와 그 값은 각 Parent의 props를 통해 전달되어야 한다. 이렇게 인터페이스에 추가함으로써 타입스크립트로 하여금 두 가지를 체크하게 하는 것이다.
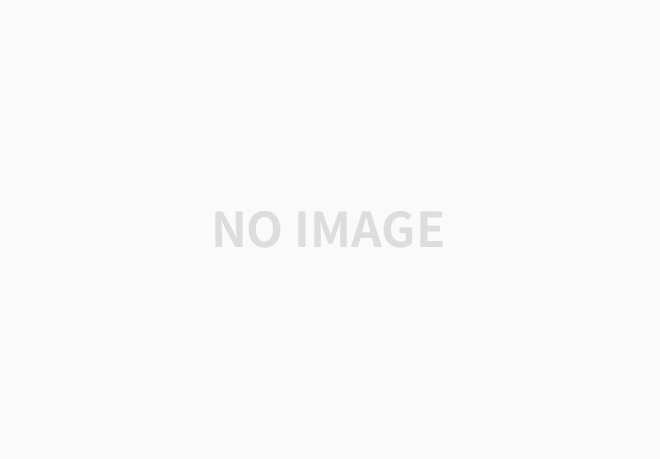
- Parent에서 볼 때 Child로 올바른 props를 전달하고 있는가?
- Child에서 정확한 이름과 타입(type)을 사용하고 있는가?
예제 코드로 만들어보자. 아래와 같이 Child 컴포넌트가 있다.
export const Child = () => {
return (
<div>
Hello!
</div>
);
};
여기에 인터페이스(interface)를 정의를 해보자. 여기서 정의한 인터페이스는 Child 컴포넌트가 받게 될 props를 정의하는 것이다.
interface ChildProps {
color: string;
}
Child는 color라는 문자열을 props로 넘길 수 있다고 정의하는 것이다. 아래와 같이 Child에서 props를 받을 수 있다.
export const Child = ({ color }: ChildProps) => {
return (
<div>
{color}
</div>
);
};
여기서 ({ color }: ChildProps)
는 (props: ChildProps)
를 비구조화 한 것이다.
ChildProps 인터페이스를 추가함으로써 타입스크립트는 Child 컴포넌트 내부에 props를 올바르게 사용하고 있는지 체크한다. color에 마우스 오버를 하면 string 값인 것을 확인할 수 있다.
Parent 컴포넌트는 아래와 같다.
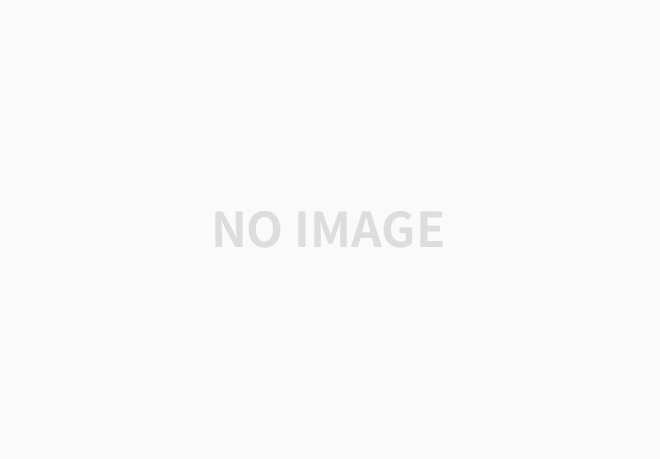
그림에서 처럼 color 프로퍼티가 빠져있다는 것을 알 수 있다. Parent에서 Child 컴포넌트를 사용할 때 color를 props로 넘겨야 한다는 것을 알 수 있다. 그래서 color를 props로 넘기면 정상적으로 동작하는 것을 알 수 있다.
const Parent = () => {
return <Child color="red" />;
};
React 18부터 Child로 넘길 때 아래와 같은 오류가 발생하는 것을 알 수 있다.
만일 Child에 children이 있는 경우 아래와 같은 오류가 발생한다.
const Parent = () => { return ( <Child color="red"> <div>content...</div> </Child> ); };
TS2322: Type { children: Element; color: string; } is not assignable to type IntrinsicAttributes & ChildProps
Property children does not exist on type IntrinsicAttributes & ChildProps[처리 방법]
Child의 interface에서
children?: React.ReactNode
을 추가해줘야 한다.
interface ChildProps { color: string; children?: React.ReactNode; }
참고
https://www.udemy.com/course/react-and-typescript-build-a-portfolio-project